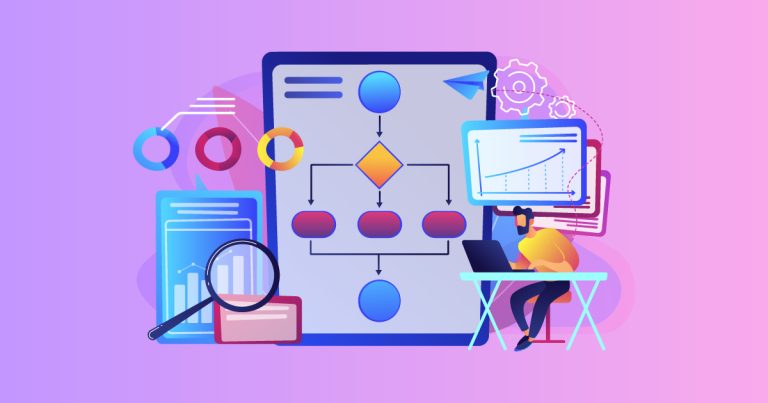
Understanding Graph Algorithms and Their Importance
In the expansive world of algorithms and data structures, graph algorithms stand out due to their ability to model and solve real-world problems in diverse domains. From social networks to transportation systems, graphs provide a natural way to represent relationships between entities. Let’s dive into the world of graph algorithms and unravel their significance.
What is a Graph?
A graph is a data structure consisting of nodes (or vertices) and edges that connect pairs of nodes. There are several types of graphs:
- Undirected Graph: Edges do not have a direction. If node A is connected to node B, B is also connected to A.
- Directed Graph (or Digraph): Edges have a direction.
- Weighted Graph: Edges have weights or costs associated with them.
Fundamental Graph Algorithms
Depth-First Search (DFS)
DFS is a traversal algorithm that dives as deep as possible from a starting node before backtracking. It’s useful for pathfinding and topological sorting.
def dfs(graph, node, visited):
if node not in visited:
print(node)
visited.add(node)
for neighbor in graph[node]:
dfs(graph, neighbor, visited)
graph = {'A': ['B', 'C'], 'B': ['D', 'E'], 'C': [], 'D': [], 'E': ['F'], 'F': []}
dfs(graph, 'A', set())
Breadth-First Search (BFS)
BFS explores all neighbor nodes at the current depth before moving on to nodes at the next depth level. It’s crucial for shortest path problems.
def bfs(graph, start):
visited, queue = set(), [start]
while queue:
vertex = queue.pop(0)
if vertex not in visited:
print(vertex)
visited.add(vertex)
queue.extend(node for node in graph[vertex] if node not in visited)
bfs(graph, 'A')
Dijkstra’s Algorithm
Used for finding the shortest path in weighted graphs.
import heapq
def dijkstra(graph, start):
shortest_path = {vertex: float('infinity') for vertex in graph}
shortest_path[start] = 0
priority_queue = [(0, start)]
while priority_queue:
current_dist, current_vertex = heapq.heappop(priority_queue)
if current_dist > shortest_path[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_dist + weight
if distance < shortest_path[neighbor]:
shortest_path[neighbor] = distance
heapq.heappush(priority_queue, (distance, neighbor))
return shortest_path
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
print(dijkstra(graph, 'A'))
Importance of Graph Algorithms
Real-World Modeling
Many real-world scenarios, such as social networks, the internet, and road systems, can be modeled as graphs, making graph algorithms essential for solving problems in these domains.
Optimization
Graph algorithms can help find the most efficient route, shortest path, or optimal network design, leading to cost savings and efficiency improvements.
Data Retrieval
In databases, graph-based algorithms can be used for faster data retrieval, especially in graph databases like Neo4j.
Recommendation Systems
Platforms like YouTube or Netflix use graph algorithms to suggest content by analyzing user behavior patterns and connections.
Graph algorithms, with their ability to model relationships and connections, offer invaluable tools in the computer scientist’s toolkit. Their versatility and applicability in real-world scenarios make them a crucial area of study for anyone delving into the world of algorithms and data structures. Whether it’s optimizing routes, designing networks, or making data-driven recommendations, graph algorithms are at the heart of many modern technological marvels.