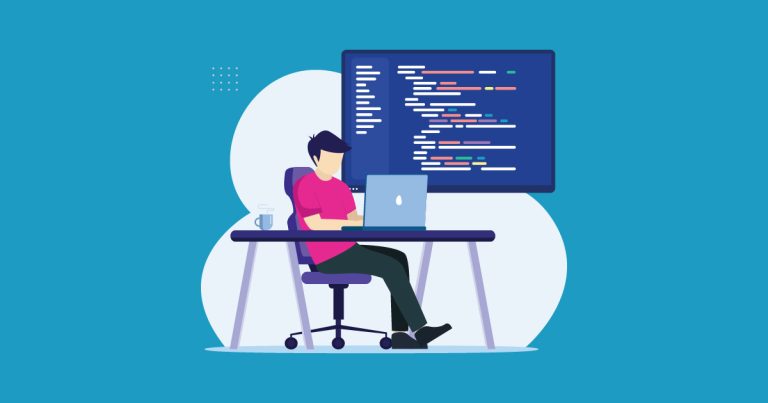
Trees and Graphs: Advanced Data Structures Explained
In the realm of computer science, data structures play a pivotal role in organizing and processing information efficiently. While arrays and linked lists lay the foundation, trees and graphs elevate our capabilities, enabling us to solve complex problems with elegance. Let’s journey through these advanced data structures and unravel their intricacies.
Trees
A tree is a hierarchical data structure that consists of nodes connected by edges. It’s a special form of a graph but without cycles.
Basic Properties:
- Each tree has a root node.
- Every node (except the root) is connected by exactly one edge from another node; this preceding node is its parent.
Types of Trees:
Binary Tree: Every node has at most two children, typically referred to as the left and right child.
Binary Search Tree (BST): It’s a binary tree with a special property: For each node, all elements in the left subtree are less than the node, and all elements in the right subtree are greater than the node.
class TreeNode:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
Balanced Trees (AVL, Red-Black Trees): These are BSTs balanced in a way to ensure O(log n) height to guarantee efficient operations.
Trie (Prefix Tree): A tree-like data structure that proves efficient for searching in a large dataset of strings. Each node of the Trie represents a single character of a string.
Graphs
A graph is a collection of nodes connected by edges. Unlike trees, graphs can have cycles.
Types of Graphs:
Undirected Graph: Edges don’t have a direction. If node A is connected to node B, then node B is also connected to node A.
Directed Graph (Digraph): Edges have a direction. If an edge goes from node A to node B, it doesn’t mean there’s an edge from node B to node A.
Weighted Graph: Each edge has a weight or cost associated with it.
Representation:
Adjacency Matrix: A 2D matrix of size V x V (V: number of vertices). A slot m[i][j]
= 1 indicates there’s an edge from vertex i to vertex j.
Adjacency List: An array of lists. The size of the array is the number of vertices. An entry m[i]
represents the list of vertices adjacent to the i-th vertex.
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F', 'G'],
'D': ['B'],
'E': ['B', 'H'],
'F': ['C'],
'G': ['C'],
'H': ['E']
}
Why Trees and Graphs?
Hierarchical Structure: Trees are ideal for scenarios with a hierarchical relationship, e.g., file systems.
Connectivity and Paths: Graphs model networks—social networks, road networks, etc. Algorithms can find the shortest path, detect cycles, or check connectivity.
Dynamic Data: Both trees (especially balanced trees like AVL) and graphs can efficiently handle insertions and deletions.
Common Algorithms:
Trees
- Traversal: In-order, Pre-order, and Post-order traversals for binary trees.
- Balancing: Rotations in AVL trees to ensure balance.
Graphs
- Depth-First Search (DFS): An algorithm to traverse or search tree or graph structures.
- Breadth-First Search (BFS): Uses a queue to traverse or search graph structures level by level.
- Dijkstra’s Algorithm: Finds the shortest path from a single source vertex to all other vertices in a weighted graph.
Trees and graphs, with their advanced structuring capabilities, offer unique solutions to challenging problems. Whether it’s organizing hierarchical data, ensuring fast lookups, or modeling networks, these data structures are indispensable tools in a programmer’s toolkit. As you delve deeper into computer science and tackle complex challenges, a solid understanding of trees and graphs will undoubtedly serve as a valuable asset.