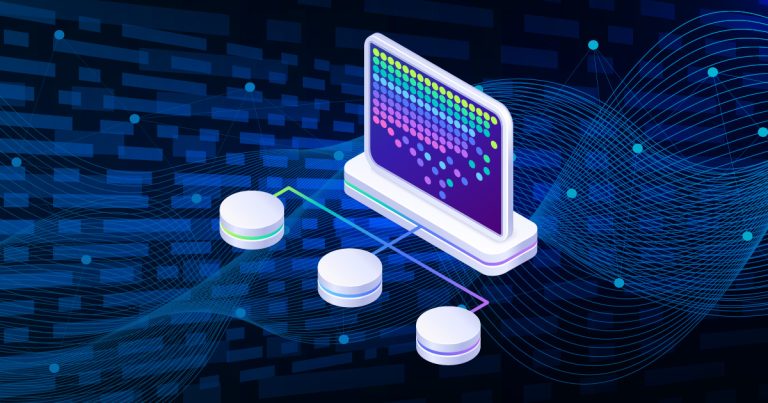
The Magic of Hashing: From Storage to Retrieval
In the vast landscape of computer science, certain concepts act as fundamental building blocks while retaining an air of mystique. Hashing, with its omnipresence in databases, cryptography, and more, is one such concept. But what is hashing, and why is it so crucial? Let’s dive deep into the world of hashing and decipher its magic.
What is Hashing?
At its essence, hashing is a process that converts input data (of any size) into a fixed-size value, usually for the purpose of fast data retrieval. Think of it as a magical box: you put in a letter, and out comes a number—always the same number for that letter, but different letters will (ideally) produce different numbers.
The Heart of Hashing: The Hash Function
The hash function is the algorithm that transforms the input data into a hash code, which can then be used as an index to store the original data. The ideal hash function has two main properties:
- It returns the hash code, which is of a fixed size.
- It’s very efficient, meaning it should return the hash code in a constant time complexity.
Applications of Hashing
Data Retrieval
One of the most common applications of hashing is in data storage and retrieval systems, like databases or caches. Items are stored by mapping them to a hash code, allowing for constant time average complexity during retrieval.
Cryptography
In the realm of security, hash functions are used to ensure data integrity. Cryptographic hash functions take input data and return a fixed-size string, which is typically a digest. Even a minor change in input will produce a vastly different digest, making it easy to detect any tampering.
Load Balancing
Hashing can distribute traffic across a number of servers or databases, ensuring that each one gets approximately the same number of requests.
The Magic Tool: Hash Tables
A hash table (or hash map) is a data structure that implements an associative array, a structure that can map keys to values. Here’s a basic example in Python:
class HashTable:
def __init__(self):
self.size = 10
self.table = [None] * self.size
def _hash(self, key):
return key % self.size
def set(self, key, value):
hash_key = self._hash(key)
self.table[hash_key] = value
def get(self, key):
hash_key = self._hash(key)
return self.table[hash_key]
Handling Collisions
Collisions occur when the hash function returns the same output for two different inputs. There are multiple ways to handle collisions:
Chaining
Each cell of the hash table points to a linked list of records that have the same hash code.
Open Addressing
When a collision occurs, we search for the next open slot in the hash table and store the item there.
The Importance of a Good Hash Function
A poorly constructed hash function can lead to clustering, where certain locations in the hash table get more keys mapped to them than others. This can significantly decrease performance. Thus, designing a good hash function is crucial to ensure uniform distribution and reduce collisions.
Dynamic Resizing
To maintain the efficiency of retrieval, the hash table can be resized dynamically based on the load factor (number of entries divided by table size). When the load factor exceeds a particular threshold, the table can be increased in size.
Hashing, while often operating behind the scenes, is an unsung hero in computer science. It facilitates rapid data storage and retrieval, bolsters security mechanisms, and streamlines computational processes. By translating vast, varied inputs into fixed, easily identifiable outputs, hashing embodies a magical blend of simplicity and efficiency, making it a cornerstone of modern computing.