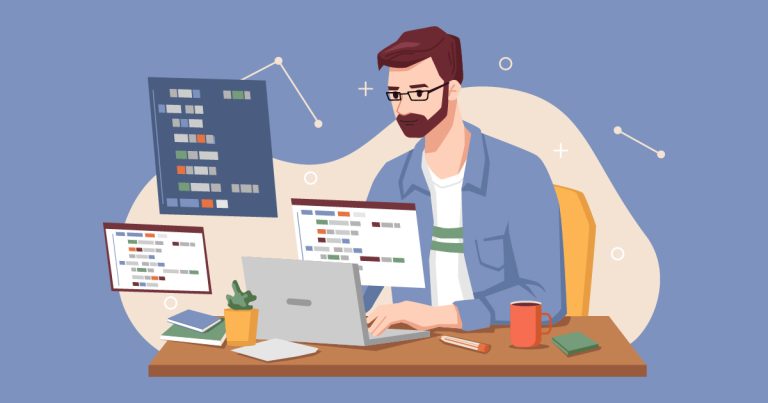
Machine Learning Algorithms: From Regression to Neural Nets
Machine learning, a subfield of artificial intelligence, has taken the world by storm. Its algorithms empower systems to make data-driven decisions and predictions. Let’s explore some popular machine learning algorithms, from basic regression models to sophisticated neural networks.
Introduction to Machine Learning
Machine learning algorithms learn patterns from data, allowing systems to make predictions or decisions without being explicitly programmed for the task. They can be broadly categorized into:
- Supervised Learning: Algorithms learn from labeled data.
- Unsupervised Learning: Algorithms learn from unlabeled data, trying to identify inherent structures.
- Reinforcement Learning: Algorithms learn by interacting with an environment and receiving feedback.
Linear Regression
Starting simple, linear regression predicts a continuous value based on one or more variables.
Example: Predicting house prices based on the number of rooms.
from sklearn.linear_model import LinearRegression
# Sample data: [number_of_rooms], price
X = [[1], [2], [3], [4]]
y = [150, 200, 250, 300]
model = LinearRegression().fit(X, y)
prediction = model.predict([[5]])
print(prediction) # Output: [350]
Decision Trees
Decision trees split data into subsets based on the value of input features. It’s a versatile algorithm used for both classification and regression tasks.
from sklearn.tree import DecisionTreeClassifier
# Sample data: [weight, height], isMale (0 for No, 1 for Yes)
X = [[70, 170], [60, 160], [80, 180], [65, 165]]
y = [1, 0, 1, 0]
model = DecisionTreeClassifier().fit(X, y)
prediction = model.predict([[72, 172]])
print(prediction) # Output: [1]
K-Means Clustering
An unsupervised learning algorithm, K-Means clustering, groups data into ‘K’ number of clusters based on feature similarity.
from sklearn.cluster import KMeans
# Sample data: [x, y] coordinates of data points
X = [[1, 2], [5, 6], [1, 3], [5, 7]]
kmeans = KMeans(n_clusters=2).fit(X)
print(kmeans.labels_) # Output: [0 1 0 1]
Neural Networks
Neural networks, inspired by the human brain’s architecture, consist of layers of interconnected nodes (neurons). They can model complex patterns and are particularly prominent in tasks like image and speech recognition.
import numpy as np
from keras.models import Sequential
from keras.layers import Dense
# Sample data
X = np.array([[0,0],[0,1],[1,0],[1,1]])
y = np.array([[0],[1],[1],[0]])
model = Sequential()
model.add(Dense(10, input_dim=2, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
model.fit(X, y, epochs=50, verbose=0)
predictions = model.predict(X)
print(predictions)
Challenges and Considerations
While machine learning algorithms can be powerful, they aren’t free from challenges:
- Overfitting: The model performs exceptionally well on training data but poorly on unseen data.
- Bias and Fairness: The algorithm’s predictions may be skewed if the training data is biased.
- Computational Complexity: Algorithms like deep learning can be resource-intensive.
Machine learning algorithms, with their diverse range and capabilities, form the backbone of many modern applications, from recommending a movie to driving a car. While this article merely scratches the surface, it underscores the transformative potential of machine learning. Whether you’re a budding data scientist or a curious observer, understanding these algorithms offers a glimpse into the future of technology.