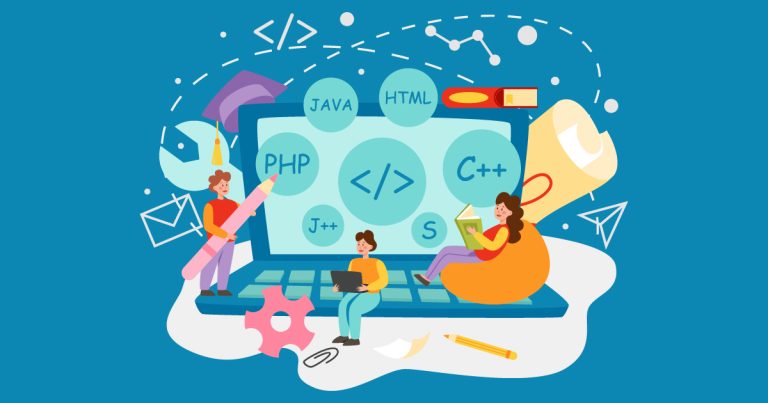
Mastering Git: Advanced Version Control Techniques
Git is a powerful version control system. While the basics can get you a long way, diving deeper into advanced techniques can make you a Git power user. This article touches upon some of these techniques.
Interactive Rebase
Interactive rebasing allows you to alter a series of commits, changing their order, editing the changes, or squashing multiple commits into one.
git rebase -i HEAD~5
This will let you interactively rebase the last five commits. Your default text editor will open with a list of commits and options for each.
Cherry Picking
Suppose you want to apply changes from a specific commit without merging an entire branch. In that case, cherry-pick
is the command to use.
git cherry-pick commit_hash
Reflog: A Safety Net
Mistakes happen. The reflog
command shows a log of where your HEAD
and branch references have been.
git reflog
To restore a state, check out the reference.
git checkout HEAD@{2}
Stashing
You can save changes that you don’t want to commit immediately with git stash
.
git stash save "description about the changes"
Apply them later:
git stash apply
Sparse Checkout
To checkout only a part of a repository:
git init new_repo
cd new_repo
git remote add origin [repo_URL]
git config core.sparseCheckout true
Then, define the directories you want:
echo "some/dir/" >> .git/info/sparse-checkout
Finally, pull from the repository:
git pull origin master
Bisect
If a bug has crept into your codebase and you’re unsure where, bisect
can help you find the problematic commit.
Start bisecting:
git bisect start
Mark the current state as bad:
git bisect bad
Then, mark a commit where you’re sure everything was okay:
git bisect good commit_hash
Git will now check out a commit halfway between the good and bad. Test the code and tell Git whether it’s good or bad. Repeat until you isolate the bug’s source.
Custom Git Aliases
For commands you use frequently, you can set up aliases:
git config --global alias.st status
Now, git st
will execute git status
.
Submodules
For maintaining external dependencies or libraries:
git submodule add [repo_URL] [path/to/submodule]
Update the submodule:
git submodule update --init --recursive
Worktrees
Instead of cloning a repository multiple times, you can create multiple working trees from the same repo.
git worktree add ../new_worktree branch_name
The Blame Game
Identify who made changes to a file line-by-line:
git blame filename
Hooks
Automate actions at certain points (e.g., before a commit). Hooks live in the .git/hooks
directory.
Git’s capabilities go far beyond basic commands like commit
and push
. By mastering advanced techniques, you can streamline your workflow, find and fix bugs more efficiently, and manage large codebases with ease. Keep practicing, and soon you’ll find these advanced commands as intuitive as the basics.