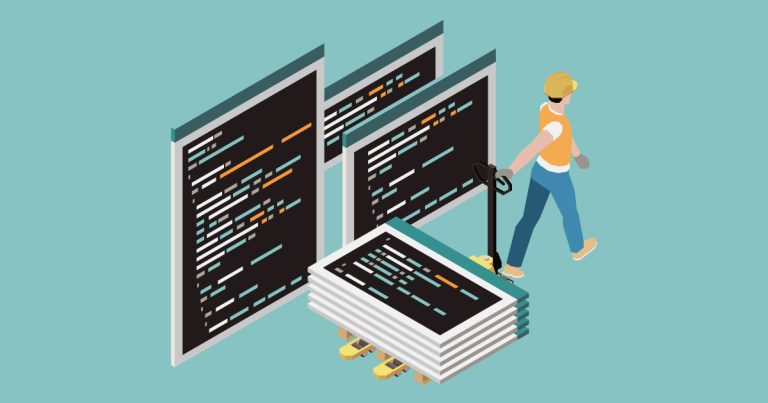
Concurrency and Parallelism in Modern Programming
Concurrency and parallelism are critical concepts in modern programming, providing opportunities to design and implement efficient, responsive, and scalable systems. This article will delve into their definitions, differences, advantages, and practical applications with code examples.
What is Concurrency?
Concurrency is a concept where several tasks are making progress, but they might not necessarily be executing simultaneously. It’s about dealing with many things at once, like a juggler keeping multiple balls in the air, even if only one ball is being touched at any given time.
Example: In Python, using the asyncio
library provides a great way to achieve concurrency.
import asyncio
async def task_one():
print('Task One is starting...')
await asyncio.sleep(2)
print('Task One is done.')
async def task_two():
print('Task Two is starting...')
await asyncio.sleep(4)
print('Task Two is done.')
asyncio.run(asyncio.gather(task_one(), task_two()))
In this example, while task_one
is sleeping, the control goes back to the event loop, allowing task_two
to run concurrently.
What is Parallelism?
Parallelism is when two or more tasks run simultaneously on separate CPU cores. It’s about doing many things at the same time.
Example: Using Python’s multiprocessing
module, we can achieve parallelism.
from multiprocessing import Process
def task_one():
print('Task One is starting...')
# Some CPU-bound operation
print('Task One is done.')
def task_two():
print('Task Two is starting...')
# Some CPU-bound operation
print('Task Two is done.')
p1 = Process(target=task_one)
p2 = Process(target=task_two)
p1.start()
p2.start()
p1.join()
p2.join()
Here, both tasks can run simultaneously on different CPU cores.
Differences between Concurrency and Parallelism
Nature: Concurrency is about dealing with many things at once, while parallelism is about doing many things at once.
Goal: Concurrency aims to handle more tasks simultaneously, improving system throughput. Parallelism aims to reduce task completion time.
Tools: Concurrency often uses constructs like async/await, coroutines, and event loops. Parallelism uses threads, processes, and parallel libraries.
Advantages
Performance: Both concurrency and parallelism can improve the performance of applications, be it I/O-bound or CPU-bound.
Responsiveness: Concurrency can help keep applications responsive by allowing non-blocking operations.
Resource Utilization: Parallelism makes better use of multi-core processors, achieving faster computations.
Challenges and Considerations
Complexity: Writing concurrent and parallel code can introduce new challenges, like race conditions, deadlocks, and more.
Testing: Concurrency issues can be non-deterministic, making them harder to reproduce and debug.
Resource Contention: With parallel execution, shared resources may become a bottleneck if not managed correctly.
Both concurrency and parallelism are essential tools in a developer’s toolkit, offering opportunities to design more efficient and responsive applications. Understanding their differences, advantages, and challenges can help developers make informed decisions when architecting systems.