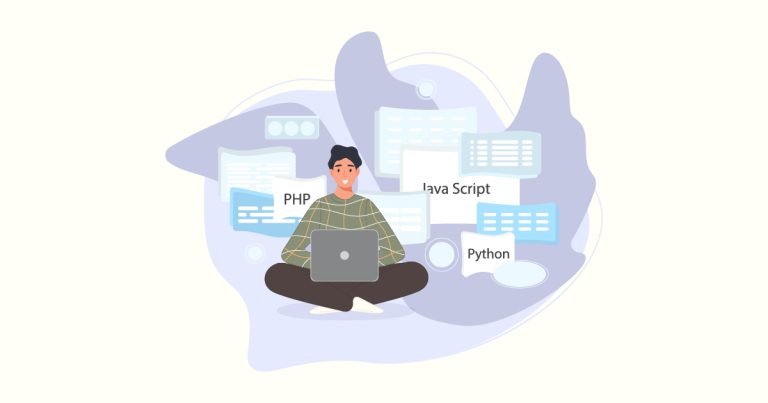
The Luhn Algorithm: A Deep Dive
The Luhn Algorithm, also known as the “modulus 10” or “mod 10” algorithm, is a simple checksum formula that has seen widespread adoption in various industries, notably for validating a variety of identification numbers. Whether you realize it or not, you likely encounter the Luhn Algorithm in your daily life, especially if you use credit cards. Here’s a closer look at this nifty mathematical technique.
History and Purpose
The Luhn Algorithm was devised by Hans Peter Luhn, an IBM scientist, in the late 1950s. The primary intent behind the algorithm was to guard against accidental errors, such as a mistyped credit card number or a misread of a magnetic stripe. It is essential to note that the Luhn Algorithm is not meant for cryptographic security or to verify the legitimacy of a number in terms of ownership, only its structural validity.
How It Works
The basic idea behind the Luhn Algorithm is quite straightforward:
- Double every second digit: Start from the rightmost digit (which is the check digit) and double the value of every second digit. If this doubling results in a number greater than 9, subtract 9 from it.
- Sum all the digits: Add together all the individual digits of the number, including both the untouched digits and the doubled digits (after they’ve been reduced by 9 if necessary).
- Modulus operation: The total sum should be divisible by 10 (i.e., modulus 10 result is 0) for the number to be valid according to the Luhn Algorithm.
An Example
Consider the number: 123456. Let’s validate it using the Luhn Algorithm:
- From the rightmost side, our digits (after doubling every second one) will look like this: 1, 4, 3, 8, 5, 12 (note that the 12 needs further processing).
- 12 becomes 1 + 2 = 3.
- Sum all the digits: 1 + 4 + 3 + 8 + 5 + 3 = 24.
- Check the modulus 10 of the sum: 24 mod 10 is 4, which is not 0. Hence, 123456 is not a valid number according to the Luhn Algorithm.
Real-world Applications
- Credit and Debit Cards: The final digit of a credit card number is a checksum that ensures the card number is valid as per the Luhn Algorithm. It helps in catching accidental misentries.
- IMEI Numbers: The International Mobile Equipment Identity number, used for identifying mobile devices, often uses the Luhn Algorithm for validation.
- National Provider Identifier: Used in the US healthcare industry, this identifier for health care providers also employs the Luhn Algorithm.
Limitations and Considerations
While the Luhn Algorithm is useful for catching innocent mistakes, it’s not foolproof. It can’t detect transpositions of adjacent numbers except if they’re 0 and 9. Moreover, it’s not designed to detect malicious alterations or provide cryptographic security.
Code Examples!
Python
def luhn_check(num):
digits = [int(d) for d in str(num)]
checksum = 0
# Double every second digit from the right
for i in range(-2, -len(digits)-1, -2):
double = digits[i] * 2
if double > 9:
double -= 9
digits[i] = double
# Check if the sum of the digits is divisible by 10
return sum(digits) % 10 == 0
# Test
print(luhn_check(123456)) # False
print(luhn_check(4111111111111111)) # True
PHP
function luhn_check($num) {
$digits = str_split($num);
$checksum = 0;
// Double every second digit from the right
for ($i = count($digits) - 2; $i >= 0; $i -= 2) {
$double = $digits[$i] * 2;
if ($double > 9) {
$double -= 9;
}
$digits[$i] = $double;
}
// Check if the sum of the digits is divisible by 10
return array_sum($digits) % 10 == 0;
}
// Test
echo luhn_check(123456) ? "True" : "False"; // False
echo luhn_check(4111111111111111) ? "True" : "False"; // True
JavaScript
function luhnCheck(num) {
let digits = Array.from(String(num), Number);
let checksum = 0;
// Double every second digit from the right
for (let i = digits.length - 2; i >= 0; i -= 2) {
let double = digits[i] * 2;
if (double > 9) {
double -= 9;
}
digits[i] = double;
}
// Check if the sum of the digits is divisible by 10
return digits.reduce((a, b) => a + b, 0) % 10 === 0;
}
// Test
console.log(luhnCheck(123456)); // false
console.log(luhnCheck(4111111111111111)); // true
These code samples validate a number using the Luhn Algorithm. The test using the number 123456
should return False
and for 4111111111111111
should return True
(or its equivalent in the respective language) for all three implementations.
The Luhn Algorithm stands as a testament to the enduring value of elegant, simple solutions in the tech world. While it might seem basic, its widespread use and efficiency in catching simple errors make it indispensable in various sectors. However, understanding its purpose and limitations is essential to ensure that it’s applied in the right context.