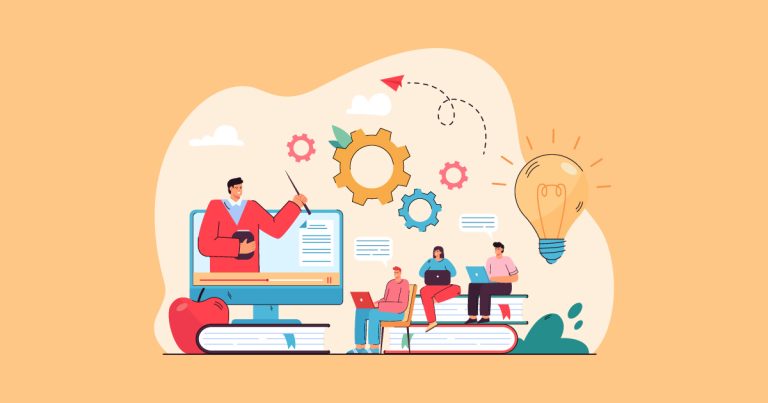
The Beauty of Recursion: Unraveling the Elegance of Repetitive Solutions
Recursion is a concept that often evokes wonder and confusion in equal measure among budding programmers. At its core, recursion is the process by which a function calls itself, either directly or indirectly, in order to solve a problem. While it may seem mystifying at first glance, recursion provides a uniquely elegant approach to solving problems that can be broken down into smaller, similar subproblems. In this article, we’ll dive deep into the beauty of recursion, understanding its essence, and exploring real-world problems that are solved more elegantly through recursive solutions.
What is Recursion?
To put it simply, recursion is when a function calls itself in its definition. Just as we have loops like for
and while
to perform repetitive tasks, recursion achieves a similar outcome but in a different, often more succinct manner. However, for recursion to be effective and not run indefinitely, there must be a base condition that stops the recursive call.
Anatomy of a Recursive Function
Every recursive function typically has two main parts:
- Base Case: This is the condition under which the function stops calling itself and starts unwinding.
- Recursive Case: This is where the function divides the problem into a smaller instance and calls itself.
The Classic Example: Factorial Calculation
The factorial of a number n, denoted as n!, is the product of all positive integers up to n. For instance, 5!=5×4×3×2×1.
Using recursion, the factorial can be computed as:
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n-1)
Here:
- Base Case: n=1, where the function returns 1.
- Recursive Case: The function calls itself with n−1.
Real-World Recursive Beauty: The Tower of Hanoi
The Tower of Hanoi is a mathematical game that consists of three rods and a number of disks of different sizes. The puzzle starts with the disks stacked on one rod in order of size, the smallest at the top. The objective is to move the entire stack to another rod, obeying the following rules:
- Only one disk can be moved at a time.
- A disk can only be moved to the top of another stack or onto an empty rod.
- No disk may be placed on top of a smaller disk.
The recursive solution elegantly breaks down the problem:
- Move n−1 disks from the source to the auxiliary peg.
- Move the nth disk from the source to the destination peg.
- Move the n−1 disks from the auxiliary peg to the destination peg, using the source peg as auxiliary.
def towerOfHanoi(n, from_rod, to_rod, aux_rod):
if n == 1:
print("Move disk 1 from rod {} to rod {}".format(from_rod, to_rod))
return
towerOfHanoi(n-1, from_rod, aux_rod, to_rod)
print("Move disk {} from rod {} to rod {}".format(n, from_rod, to_rod))
towerOfHanoi(n-1, aux_rod, to_rod, from_rod)
n = 3 # Number of disks
towerOfHanoi(n, 'A', 'C', 'B') # Names of the rods
In this example, the function towerOfHanoi
uses recursion to determine the steps needed to solve the puzzle. It breaks down the problem by first moving n-1
disks to the auxiliary rod, then moving the nth disk to the destination rod, and finally moving the n-1
disks from the auxiliary rod to the destination rod using the source rod as auxiliary.
Why Recursion?
Recursion offers a few key advantages:
- Simplicity: Recursive solutions are often more concise and easier to understand.
- Problem Decomposition: It allows for natural breakdown of problems into similar sub-problems.
However, it’s worth noting that recursion might not always be the most efficient solution. Excessive recursive calls can lead to a stack overflow.
Recursion is a testament to the beauty and depth of computer science. It stands as a reminder that sometimes, solutions don’t always have to be about moving forward—sometimes, they involve delving deeper into the problem itself, only to emerge with a clearer solution. As you advance in your coding journey, challenge yourself to think recursively, and you might find that the most elegant solution was hidden within the problem all along.
Whether you’re a seasoned developer or just starting out, diving into recursion can open up a world of elegant problem-solving techniques that are both challenging and rewarding.