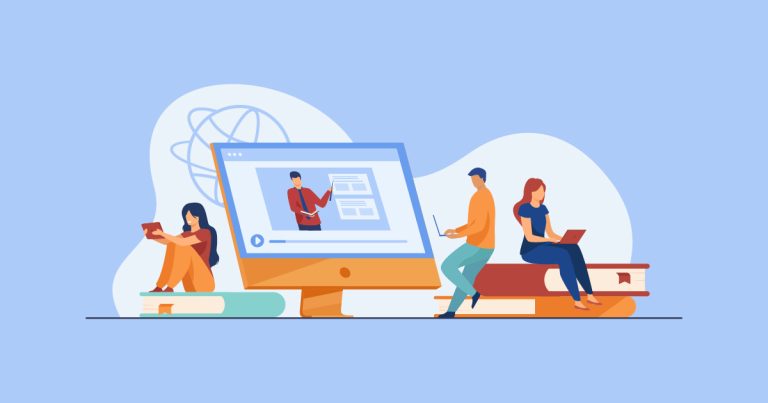
Sorting Algorithms Part 3: Efficiency (3 Part Series)
In the realm of computer science, the efficacy of sorting algorithms isn’t just about whether they can arrange data in a particular order, but how optimally they do so. This “Efficiency” section delves deep into this critical aspect, providing readers with a comprehensive understanding of what makes one sorting algorithm stand out from another. Here, we’ll unravel the nuances of time and space complexities, shedding light on their implications in real-world scenarios. Additionally, we’ll touch upon other crucial factors like stability and adaptivity, offering a holistic view of sorting algorithm performance. Dive in to decipher the intricacies that dictate the speed and resourcefulness of these fundamental algorithms.
The efficiency of sorting algorithms can be determined by analyzing their performance in various aspects, including but not limited to:
Time Complexity: This quantifies the amount of time an algorithm takes based on the length of the input. The most important complexities to consider are:
- Worst-case: The maximum number of operations executed for any input of size n.
- Average-case: The expected number of operations for a random input of size n.
- Best-case: The minimum number of operations executed for any input of size n.
Space Complexity: This quantifies the amount of additional memory the algorithm uses apart from the input data.
Stability: If the relative order of equal elements remains unchanged in the sorted output, the algorithm is considered stable.
Adaptivity: If an algorithm performs better when dealing with partially sorted data compared to completely random data, it’s adaptive.
Given these criteria, let’s analyze the algorithms, we will use Big O notation to do so. In computer science, the Big O notation, often represented as “O(…)”, provides a high-level measure of an algorithm’s efficiency by describing its worst-case or upper bound performance. Specifically, it quantifies how the running time or space requirements of an algorithm grow relative to the input size, abstracting away constant factors and lower-order terms. This notation offers a way to categorize algorithms by their scalability, helping developers anticipate how an algorithm might perform as input sizes increase, and thus make informed decisions when choosing between different algorithms for a specific task.
- Bubble Sort:
- Time Complexity:
- Best-case: O(n) – when the array is already sorted.
- Average-case: O(n2)
- Worst-case: O(n2)
- Space Complexity: O(1) – in-place sorting.
- Stability: Stable
- Time Complexity:
- Selection Sort:
- Time Complexity:
- Best-case: O(n2)
- Average-case: O(n2)
- Worst-case: O(n2)
- Space Complexity: O(1) – in-place sorting.
- Stability: Unstable
- Time Complexity:
- Insertion Sort:
- Time Complexity:
- Best-case: O(n) – when the array is already sorted.
- Average-case: O(n2)
- Worst-case: O(n2)
- Space Complexity: O(1) – in-place sorting.
- Stability: Stable
- Time Complexity:
- Merge Sort:
- Time Complexity:
- Best-case: O(n log n)
- Average-case: O(n log n)
- Worst-case: O(n log n)
- Space Complexity: O(n)
- Stability: Stable
- Time Complexity:
- Quick Sort:
- Time Complexity:
- Best-case: O(n log n) – when a good pivot is chosen.
- Average-case: O(n log n)
- Worst-case: O(n2) – when a bad pivot is chosen, e.g., sorted or reverse sorted array.
- Space Complexity: O(log n)
- Stability: Unstable
- Time Complexity:
- Heap Sort:
- Time Complexity:
- Best-case: O(n log n)
- Average-case: O(n log n)
- Worst-case: O(n log n)
- Space Complexity: O(1)
- Stability: Unstable
- Time Complexity:
- Radix Sort:
- Time Complexity:
- Best-case: O(nk) – where k is the number of digits of the maximum number.
- Average-case: O(nk)
- Worst-case: O(nk)
- Space Complexity: O(n+k)
- Stability: Stable
- Time Complexity:
In real-world applications, the efficiency of an algorithm also depends on various other factors, such as data distribution, architectural details of the system on which the algorithm runs, cache sizes, and more. As such, theoretical analysis should be supplemented with empirical testing to determine the most suitable sorting algorithm for a particular application.