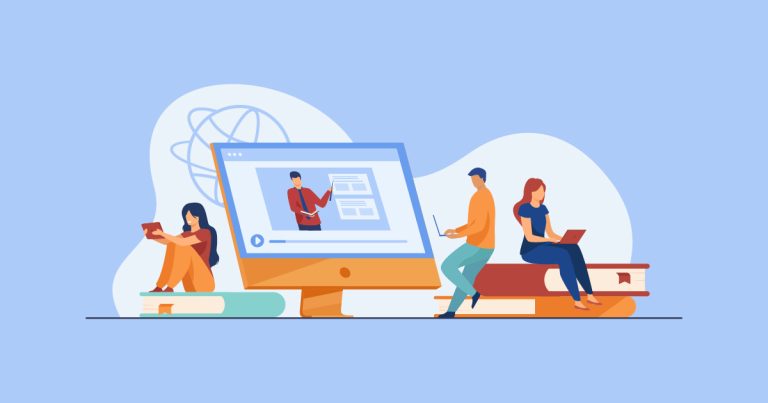
Sorting Algorithms Part 1: An Overview (3 Part Series)
Sorting algorithms are fundamental to computer science, and they play a crucial role in various applications, ranging from simple data organization tasks to the backbone of more complex algorithms.
Definition
A sorting algorithm is a method to rearrange a sequence of items in a particular order – often numerical or lexicographical. The sequence could be an array, list, or any data structure that supports the required operations.
Types of Sorting Algorithms
- Bubble Sort: It repeatedly steps through the list, compares adjacent items and swaps them if they’re in the wrong order. The process is repeated until the list is sorted.
- Selection Sort: This algorithm divides the input list into two parts: a sorted section and an unsorted section, then repeatedly selects the smallest (or largest) element from the unsorted section and moves it to the end of the sorted section.
- Insertion Sort: Like Selection Sort, it divides the list into sorted and unsorted sections, but it builds the sorted section by moving one element at a time to its correct position.
- Merge Sort: A divide and conquer algorithm that splits the list in half, recursively sorts both halves, and then merges them back together.
- Quick Sort: Another divide and conquer method. It selects a ‘pivot’ element and partitions the array around the pivot, placing all smaller elements before and all larger elements after the pivot. Then it recursively sorts the partitions.
- Heap Sort: Uses a binary heap data structure to first build a ‘heap’ from the input data, then repeatedly extracts the maximum element and reconstructs the heap until it’s empty.
- Radix Sort: A non-comparative sort that processes individual digits of numbers (or letters in strings) to sort a list, typically starting from the least significant digit (or letter) and moving to the most significant.
Factors to Consider When Choosing a Sorting Algorithm
- Stability: Does the algorithm maintain the relative order of equal elements?
- Adaptive: Does the algorithm benefit from the pre-ordering of items?
- Memory: How much extra memory does the algorithm require?
- Number of Comparisons/Swaps: For larger datasets, fewer comparisons or swaps can make a significant difference in performance.
- Worst, Average, and Best Case Performance: Some algorithms may have excellent average-case performance but terrible worst-case performance.
Applications
- Databases: Many database operations, like ORDER BY clauses, are underpinned by sorting algorithms.
- Search Algorithms: Efficient search algorithms, like binary search, require data to be sorted.
- Graphics: Algorithms that render scenes often require sorting, for example in the Painter’s algorithm where surfaces are drawn in order from farthest to closest.
While sorting may seem like a mundane task, the efficiency of a sorting algorithm can have far-reaching impacts on system performance. Understanding the intricacies and applications of different sorting algorithms equips developers to make informed decisions, optimizing both performance and resources.
In tomorrow’s post (Part 2 of 3) we will take a deeper dive into each type of sorting algorithm with code examples on how to implement them in python.