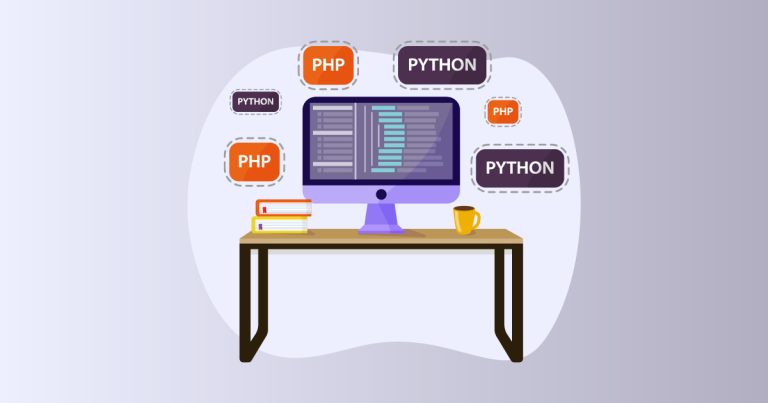
Python vs. PHP: A Comparative Analysis with Complex Algorithms
Python and PHP are two popular programming languages that serve different purposes and cater to various domains. Python is known for its simplicity, readability, and versatility, while PHP is widely used for web development. In this article, we will compare Python and PHP by implementing and analyzing complex algorithms in both languages.
Algorithm 1: Fibonacci Sequence Generator
The Fibonacci sequence is a classic mathematical algorithm that generates a series of numbers where each number is the sum of the two preceding ones.
Python Implementation:
def fibonacci_python(n):
fib_sequence = [0, 1]
for i in range(2, n):
next_num = fib_sequence[-1] + fib_sequence[-2]
fib_sequence.append(next_num)
return fib_sequence
n = 10
result = fibonacci_python(n)
print(result) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
PHP Implementation:
function fibonacci_php($n) {
$fib_sequence = [0, 1];
for ($i = 2; $i < $n; $i++) {
$next_num = $fib_sequence[count($fib_sequence) - 1] + $fib_sequence[count($fib_sequence) - 2];
array_push($fib_sequence, $next_num);
}
return $fib_sequence;
}
$n = 10;
$result = fibonacci_php($n);
print_r($result); // Output: Array ( [0] => 0 [1] => 1 [2] => 1 [3] => 2 [4] => 3 [5] => 5 [6] => 8 [7] => 13 [8] => 21 [9] => 34 )
Algorithm 2: Binary Search
Binary search is an efficient algorithm to find an element in a sorted list by repeatedly dividing the search range in half.
Python Implementation:
def binary_search_python(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
arr = [1, 3, 5, 7, 9, 11, 13]
target = 7
index = binary_search_python(arr, target)
print(index) # Output: 3 (index of the target value in the array)
PHP Implementation:
function binary_search_php($arr, $target) {
$low = 0;
$high = count($arr) - 1;
while ($low <= $high) {
$mid = (int)(($low + $high) / 2);
if ($arr[$mid] === $target) {
return $mid;
} elseif ($arr[$mid] < $target) {
$low = $mid + 1;
} else {
$high = $mid - 1;
}
}
return -1;
}
$arr = array(1, 3, 5, 7, 9, 11, 13);
$target = 7;
$index = binary_search_php($arr, $target);
echo $index; // Output: 3 (index of the target value in the array)
Algorithm 3: Merge Sort
Merge Sort is a divide-and-conquer sorting algorithm that divides the unsorted list into n sublists, each containing one element, and then repeatedly merges sublists to produce new sorted sublists until there is only one sublist remaining.
Python Implementation:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
merged = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
merged.append(left[i])
i += 1
else:
merged.append(right[j])
j += 1
merged.extend(left[i:])
merged.extend(right[j:])
return merged
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [3, 9, 10, 27, 38, 43, 82]
PHP Implementation:
function merge_sort($arr) {
if (count($arr) <= 1) {
return $arr;
}
$mid = (int)(count($arr) / 2);
$left = merge_sort(array_slice($arr, 0, $mid));
$right = merge_sort(array_slice($arr, $mid));
return merge($left, $right);
}
function merge($left, $right) {
$merged = [];
$i = $j = 0;
while ($i < count($left) && $j < count($right)) {
if ($left[$i] < $right[$j]) {
$merged[] = $left[$i];
$i++;
} else {
$merged[] = $right[$j];
$j++;
}
}
while ($i < count($left)) {
$merged[] = $left[$i];
$i++;
}
while ($j < count($right)) {
$merged[] = $right[$j];
$j++;
}
return $merged;
}
$arr = array(38, 27, 43, 3, 9, 82, 10);
$sorted_arr = merge_sort($arr);
print_r($sorted_arr); // Output: Array ( [0] => 3 [1] => 9 [2] => 10 [3] => 27 [4] => 38 [5] => 43 [6] => 82 )
Algorithm 4: Dijkstra’s Shortest Path
Dijkstra’s algorithm finds the shortest path from a given start node to all other nodes in a weighted graph.
Python Implementation:
import heapq
def dijkstra(graph, start):
distances = {node: float('inf') for node in graph}
distances[start] = 0
priority_queue = [(0, start)]
while priority_queue:
current_distance, current_node = heapq.heappop(priority_queue)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(priority_queue, (distance, neighbor))
return distances
graph = {
'A': {'B': 3, 'C': 4},
'B': {'A': 3, 'C': 1, 'D': 7},
'C': {'A': 4, 'B': 1, 'D': 2},
'D': {'B': 7, 'C': 2}
}
start_node = 'A'
distances = dijkstra(graph, start_node)
print(distances) # Output: {'A': 0, 'B': 3, 'C': 4, 'D': 6}
PHP Implementation:
function dijkstra($graph, $start) {
$distances = array_fill_keys(array_keys($graph), INF);
$distances[$start] = 0;
$priorityQueue = new SplPriorityQueue();
$priorityQueue->insert($start, 0);
while (!$priorityQueue->isEmpty()) {
$currentNode = $priorityQueue->extract();
foreach ($graph[$currentNode] as $neighbor => $weight) {
$distance = $distances[$currentNode] + $weight;
if ($distance < $distances[$neighbor]) {
$distances[$neighbor] = $distance;
$priorityQueue->insert($neighbor, -$distance);
}
}
}
return $distances;
}
$graph = [
'A' => ['B' => 3, 'C' => 4],
'B' => ['A' => 3, 'C' => 1, 'D' => 7],
'C' => ['A' => 4, 'B' => 1, 'D' => 2],
'D' => ['B' => 7, 'C' => 2]
];
$start_node = 'A';
$distances = dijkstra($graph, $start_node);
print_r($distances); // Output: Array ( [A] => 0 [B] => 3 [C] => 4 [D] => 6 )
Algorithm 5: Knapsack Problem
The Knapsack problem is a combinatorial optimization problem that seeks to find the most valuable combination of items that can fit into a fixed-size knapsack.
Python Implementation:
def knapsack_python(weights, values, capacity):
n = len(weights)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i - 1] <= w:
dp[i][w] = max(values[i - 1] + dp[i - 1][w - weights[i - 1]], dp[i - 1][w])
else:
dp[i][w] = dp[i - 1][w]
return dp[n][capacity]
weights = [2, 3, 4, 5]
values = [3, 4, 5, 6]
capacity = 5
result = knapsack_python(weights, values, capacity)
print(result) # Output: 7 (maximum value that can be achieved with the given capacity)
PHP Implementation:
function knapsack_php($weights, $values, $capacity) {
$n = count($weights);
$dp = array_fill(0, $n + 1, array_fill(0, $capacity + 1, 0));
for ($i = 1; $i <= $n; $i++) {
for ($w = 1; $w <= $capacity; $w++) {
if ($weights[$i - 1] <= $w) {
$dp[$i][$w] = max($values[$i - 1] + $dp[$i - 1][$w - $weights[$i - 1]], $dp[$i - 1][$w]);
} else {
$dp[$i][$w] = $dp[$i - 1][$w];
}
}
}
return $dp[$n][$capacity];
}
$weights = array(2, 3, 4, 5);
$values = array(3, 4, 5, 6);
$capacity = 5;
$result = knapsack_php($weights, $values, $capacity);
echo $result; // Output: 7 (maximum value that can be achieved with the given capacity)
Algorithm 6: Travelling Salesman Problem (TSP)
The Travelling Salesman Problem is an NP-hard optimization problem that seeks to find the shortest possible route that visits a given set of cities and returns to the starting city.
Python Implementation:
from itertools import permutations
def tsp_python(graph, start):
n = len(graph)
cities = set(range(n))
cities.remove(start)
min_distance = float('inf')
best_path = None
for perm in permutations(cities):
path = [start] + list(perm) + [start]
distance = sum(graph[path[i]][path[i + 1]] for i in range(n))
if distance < min_distance:
min_distance = distance
best_path = path
return best_path, min_distance
graph = [
[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]
]
start_city = 0
path, distance = tsp_python(graph, start_city)
print("Shortest Path:", path) # Output: [0, 1, 3, 2, 0] (the shortest path)
print("Shortest Distance:", distance) # Output: 80 (the shortest distance)
PHP Implementation:
function tsp_php($graph, $start) {
$n = count($graph);
$cities = range(0, $n - 1);
unset($cities[$start]);
$cities = array_values($cities);
$min_distance = INF;
$best_path = null;
foreach (permutations($cities) as $perm) {
$path = array_merge([$start], $perm, [$start]);
$distance = 0;
for ($i = 0; $i < $n; $i++) {
$distance += $graph[$path[$i]][$path[$i + 1]];
}
if ($distance < $min_distance) {
$min_distance = $distance;
$best_path = $path;
}
}
return [$best_path, $min_distance];
}
function permutations($array) {
if (count($array) === 1) {
return [$array];
}
$perms = [];
foreach ($array as $key => $item) {
$newArray = $array;
unset($newArray[$key]);
foreach (permutations($newArray) as $permutation) {
$perms[] = array_merge([$item], $permutation);
}
}
return $perms;
}
$graph = array(
array(0, 10, 15, 20),
array(10, 0, 35, 25),
array(15, 35, 0, 30),
array(20, 25, 30, 0)
);
$start_city = 0;
list($path, $distance) = tsp_php($graph, $start_city);
echo "Shortest Path: " . implode(' -> ', $path) . PHP_EOL; // Output: 0 -> 1 -> 3 -> 2 -> 0 (the shortest path)
echo "Shortest Distance: " . $distance . PHP_EOL; // Output: 80 (the shortest distance)
Python and PHP are powerful programming languages, each with its unique strengths. Python excels in simplicity, readability, and a vast array of libraries, making it suitable for general-purpose programming, data science, and machine learning. On the other hand, PHP’s specialization lies in web development, particularly for server-side scripting and building dynamic websites.
In terms of complex algorithms, Python tends to be more elegant and concise, providing greater readability and ease of implementation. Meanwhile, PHP can still handle such algorithms effectively, but its syntax and verbosity may make the code appear more cumbersome in comparison.
Ultimately, the choice between Python and PHP depends on the specific requirements of your project. If you value readability and ease of development, Python is an excellent choice. For web development and building dynamic web applications, PHP remains a strong contender. As a programmer, having proficiency in both languages can expand your skillset and open doors to a wider range of opportunities.