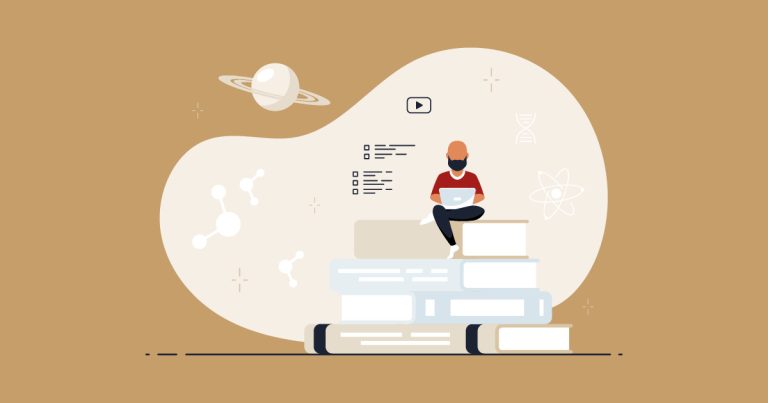
Python for PHP Programmers – A Gentle Introduction
Welcome to the world of Python! As a PHP programmer, you already possess valuable programming skills, and now you’re about to embark on a journey to explore the versatility and simplicity of Python. This lesson aims to introduce you to Python’s syntax, data structures, and core concepts, while highlighting the key differences and similarities between Python and PHP. Let’s dive in!
Python Basics and Syntax
Hello, World!
Like PHP, Python is an interpreted language. Let’s begin by writing a simple “Hello, World!” program to get started:
print("Hello, World!")
Indentation Matters
Unlike PHP, where curly braces {}
define code blocks, Python uses indentation to indicate the scope of blocks. This makes Python code clean and readable. For example:
if 5 > 2:
print("Five is greater than two!")
Variables and Data Types
Variable Declaration
In Python, you don’t need to specify the data type of a variable explicitly. Python infers the data type based on the assigned value:
message = "Hello, Python!" # message is a string
count = 42 # count is an integer
pi = 3.14 # pi is a float
Common Data Types
Python shares common data types with PHP, such as integers, floats, strings, booleans, lists, and dictionaries.
# List
fruits = ["apple", "banana", "orange"]
# Dictionary
person = {"name": "John", "age": 30, "city": "New York"}
Control Flow and Loops
If-else Statements
The syntax of conditional statements in Python resembles PHP, but remember the importance of indentation:
if condition:
# code block
elif condition:
# code block
else:
# code block
Loops
Python offers both for
and while
loops, similar to PHP:
# For loop
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
# While loop
count = 0
while count < 5:
print(count)
count += 1
Functions and Modules
Defining Functions
In Python, functions are defined using the def
keyword:
def greet(name):
return "Hello, " + name + "!"
Importing Modules
Like PHP, Python offers modules to extend its functionality. Import modules using the import
keyword:
import math
print(math.sqrt(25)) # Output: 5.0
Key Differences and Advantages of Python
Significant Whitespace
The primary difference between PHP and Python is Python’s use of significant whitespace. Embrace this feature as it leads to more readable and maintainable code.
Rich Library Ecosystem
Python’s extensive library ecosystem offers solutions for various tasks, such as data analysis, web development, and more. Explore libraries like NumPy, Pandas, and Flask to expand your skillset.
Congratulations! You have taken your first steps into the world of Python. With its clear and concise syntax, Python offers PHP programmers a new and powerful tool in their programming toolkit. As you continue your Python journey, keep practicing, exploring the vast library ecosystem, and enjoy the simplicity and elegance that Python brings to your coding endeavors.