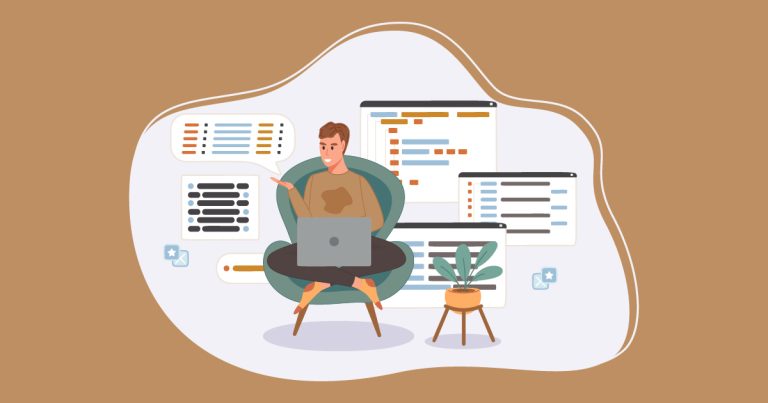
JavaScript Classes: A Comprehensive Tutorial
In JavaScript, classes provide a convenient and organized way to create objects with similar properties and behaviors. Classes were introduced in ECMAScript 6 (ES6) and offer a more structured and object-oriented approach to building applications. In this tutorial, we’ll explore JavaScript classes in detail, covering their syntax, usage, inheritance, and more.
Introduction to Classes
A class in JavaScript is a blueprint for creating objects that share common properties and methods. It encapsulates data and functionality into a single unit, making code more organized, maintainable, and reusable. Classes act as templates from which you can create instances, also known as objects.
Creating Classes
To define a class, we use the class
keyword followed by the class name. Let’s create a simple Person
class:
class Person {
// Class methods and properties will go here
}
Class Constructors
Constructors are special methods that get executed when a new instance of the class is created. They are responsible for initializing the object’s properties. You define the constructor method using the constructor
keyword:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
Class Methods
Methods are functions defined within the class and are used to perform actions or calculations related to the class. They can be accessed through class instances. Let’s add a method greet
to the Person
class:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name}, and I am ${this.age} years old.`);
}
}
Class Properties
Properties are variables that hold the state of the object. They are defined within the class and can be accessed and modified using class instances. Let’s add a property gender
to the Person
class:
class Person {
constructor(name, age, gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
greet() {
console.log(`Hello, my name is ${this.name}, and I am ${this.age} years old.`);
}
}
Static Methods
Static methods belong to the class itself and not to its instances. They are called on the class itself, rather than on an instance. Static methods are useful for utility functions that don’t depend on the instance’s state. To define a static method, use the static
keyword:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
static sayHello() {
console.log("Hello from the Person class!");
}
}
Inheritance
Inheritance is the process of creating a new class (subclass) based on an existing class (superclass). The subclass inherits the properties and methods of the superclass and can also have its own additional properties and methods. To define a subclass, use the extends
keyword:
class Student extends Person {
constructor(name, age, gender, grade) {
super(name, age, gender);
this.grade = grade;
}
study() {
console.log(`${this.name} is studying hard to achieve good grades.`);
}
}
Getters and Setters
Getters and setters allow us to control access to the properties of the class. Getters are used to retrieve the value of a property, while setters are used to set or modify the value of a property. They are defined using the get
and set
keywords, respectively:
class Person {
constructor(name, age) {
this._name = name;
this._age = age;
}
get name() {
return this._name;
}
set name(newName) {
this._name = newName;
}
}
Class Modifiers
Class modifiers like public
, private
, and protected
define the visibility and accessibility of class members. In JavaScript, these modifiers are not part of the language itself, but you can achieve similar behavior using conventions and features like closures.
JavaScript classes provide a more structured way to define and organize objects in your code. They promote code reusability, maintainability, and encapsulation. With classes, you can create blueprints for objects, define methods and properties, and even create class hierarchies using inheritance. Understanding classes is essential for developing modern and efficient JavaScript applications.
Remember that JavaScript classes are a syntactic sugar on top of the prototype-based inheritance system that JavaScript inherently uses. Under the hood, classes are still implemented using prototypes.