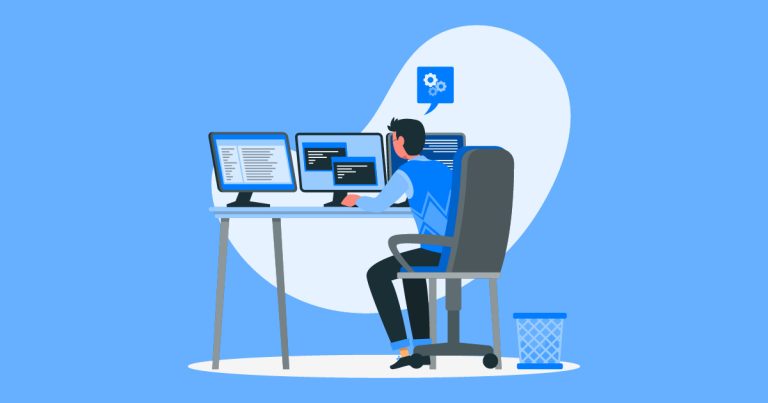
Cryptographic Puzzles and Computational Theory: Foundations of Secure Communication
In the vast domain of computational theory, cryptographic puzzles stand out as a unique intersection of mathematical intrigue and practical application. They form the bedrock of modern cryptographic systems, ensuring the confidentiality, authenticity, and integrity of our digital communications. But how do these puzzles work, and what makes them so crucial in the digital age? Let’s decrypt this mystery.
The Essence of Cryptographic Puzzles
At its core, a cryptographic puzzle is a problem that is deliberately designed to be challenging and time-consuming to solve. The goal is to introduce a delay or computational cost, ensuring that only those with the appropriate resources or keys can solve it in a reasonable amount of time.
A Historical Perspective
The concept of using puzzles and challenges for security isn’t new. The legendary story of the Gordian Knot, an intricate puzzle that Alexander the Great reportedly “solved” with a swift sword strike, serves as an early metaphor. In the realm of digital communication, though, these puzzles become gatekeepers, ensuring that only authorized entities can access or modify the data.
The Computational Theory Behind the Puzzles
Central to the effectiveness of cryptographic puzzles is the idea of computational hardness. Problems like factoring large numbers or computing discrete logarithms are believed to be “hard” for classical computers, especially when the numbers involved are massive.
This is where computational theory steps in. The belief that certain problems are inherently difficult to solve, even with powerful computers, forms the cornerstone of many cryptographic systems. If an adversary can’t solve the puzzle quickly, our data remains secure.
Applications in Modern Cryptography
- Proof-of-Work: Famously used in cryptocurrencies like Bitcoin, Proof-of-Work requires nodes in a network to solve a complex puzzle to add transactions to the blockchain. This ensures security and deters spam or denial-of-service attacks.
- Public Key Cryptosystems: Systems like RSA rely on the difficulty of factoring large numbers. While multiplying two prime numbers is easy, factoring the result back into those primes is computationally challenging, thus providing security.
- Key Derivation Functions: These functions take a password and produce a cryptographic key after applying a computationally intensive process. It ensures that brute-forcing passwords is slow and costly for attackers.
Future Prospects and Quantum Computing
While cryptographic puzzles have served us well, the advent of quantum computing poses new challenges. Quantum computers have the potential to solve certain problems, like integer factorization, exponentially faster than classical computers. This evolution pushes cryptographers to develop new puzzles and cryptographic systems that are quantum-resistant.
Code Example!
To align with the topic of cryptographic puzzles and their applications, I’ll provide a simple example of how the RSA algorithm (a Public Key Cryptosystem) works. Remember, this will be a basic illustration suitable for understanding the concepts rather than a production-level implementation.
RSA Algorithm: A Basic Overview
- Key Generation:
- Choose two prime numbers, p and q.
- Compute n=p×q and ϕ(n)=(p−1)×(q−1).
- Choose an integer e such that 1 < e < ϕ(n) and gcd(e,ϕ(n)) = 1; e is the public key.
- Compute d to satisfy the congruence relation d × e ≡ 1 mod ϕ(n); d is the private key.
- Encryption:
- Given a plaintext message M, the ciphertext C is computed as:
C = Me mod n
- Given a plaintext message M, the ciphertext C is computed as:
- Decryption:
- Using the private key d, the original message M can be found as:
M = Cd mod n
- Using the private key d, the original message M can be found as:
Python Code Example
This is a rudimentary example without optimizations or necessary security measures:
def gcd(a, b):
while b != 0:
a, b = b, a % b
return a
def modinv(e, phi):
# Extended Euclidean Algorithm for Modular Inverse
m0 = phi
y = 0
x = 1
while e > 1:
q = e // phi
t = phi
phi = e % phi
e = t
t = y
y = x - q * y
x = t
if x < 0:
x = x + m0
return x
# Key generation
p, q = 61, 53 # Example prime numbers
n = p * q
phi = (p-1) * (q-1)
# Let's set e manually to 17 for this example
e = 17
if gcd(e, phi) != 1:
print "e is not coprime to phi!"
exit(0)
d = modinv(e, phi)
# Encryption & Decryption
M = 20 # Example message
C = pow(M, e, n)
Decrypted_M = pow(C, d, n)
print "Original Message:", M
print "Encrypted:", C
print "Decrypted:", Decrypted_M
Remember, real-world cryptographic libraries have optimized and secured these algorithms for practical use. This code is for educational purposes and to illustrate the underlying concepts.
Cryptographic puzzles, rooted in the principles of computational theory, are more than mere academic curiosities. They are the sentinels of our digital world, safeguarding our data and communications against prying eyes. As technology evolves and new challenges emerge, the dance between cryptographers and adversaries continues, ensuring that the realm of secure communication remains both vibrant and robust.