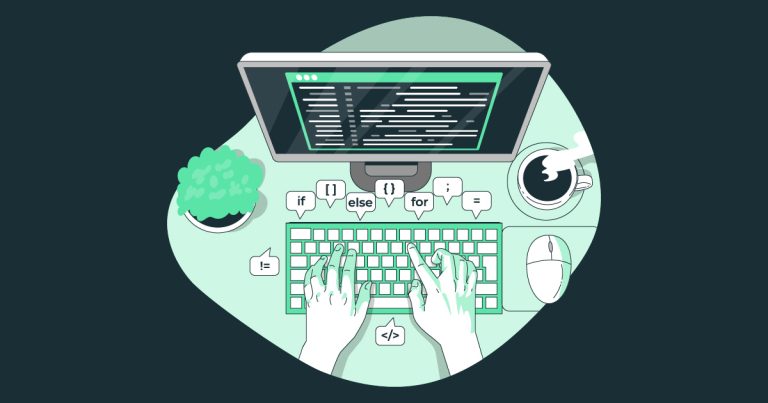
A Journey Through Programming Languages: Assembly, C/C++, and Python
Programming languages are the backbone of modern software development, enabling developers to transform ideas into functional and efficient programs. Among the plethora of languages available, three distinctive languages stand out for their varying levels of abstraction and complexity: Assembly, C/C++, and Python. In this article, we will explore these languages through a simple example code to understand their differences and use cases.
Example Code – Sum of an Array:
For our comparative study, let’s take a common programming task: finding the sum of elements in an array. We will write the same functionality in Assembly, C/C++, and Python.
Assembly Language
Assembly language is a low-level language that directly interacts with the hardware. It uses mnemonic codes to represent machine instructions and operates with registers and memory locations.
section .data
array db 1, 2, 3, 4, 5
array_size equ $ - array
section .text
global _start
_start:
xor eax, eax ; Clear register to store sum
mov ecx, array_size ; Counter to iterate through array
mov esi, array ; Address of the array
sum_loop:
add eax, [esi] ; Add the element to the sum
add esi, 1 ; Move to the next element
loop sum_loop ; Loop until the counter becomes 0
; The result (sum) is now stored in eax
; Further actions can be taken here
C/C++
C/C++ is a widely used high-level language, known for its efficiency and direct access to memory. It provides more abstraction compared to Assembly and is commonly used in systems programming and performance-critical applications.
#include <stdio.h>
int main() {
int array[] = {1, 2, 3, 4, 5};
int array_size = sizeof(array) / sizeof(array[0]);
int sum = 0;
for (int i = 0; i < array_size; i++) {
sum += array[i];
}
// 'sum' now contains the sum of the elements
// Further actions can be taken here
return 0;
}
Python
Python, on the other hand, is a high-level, interpreted language known for its simplicity and readability. It abstracts away many low-level details, making it more beginner-friendly and easier to write code.
def sum_array(array):
return sum(array)
# Usage example:
array = [1, 2, 3, 4, 5]
result = sum_array(array)
# 'result' now contains the sum of the elements
# Further actions can be taken here
Comparison
- Readability and Complexity:
- Assembly: Low-level and complex due to direct hardware interaction.
- C/C++: Moderate complexity with a balance of high-level abstractions and direct memory access.
- Python: Highly readable and simple with a focus on readability and ease of use.
- Efficiency and Performance:
- Assembly: Offers the best performance since it deals directly with the hardware. Ideal for resource-constrained environments.
- C/C++: Provides excellent performance with the ability to optimize code for efficiency.
- Python: Generally slower than the former two due to its interpreted nature, but it excels in development speed and ease of use.
- Portability:
- Assembly: Least portable, as code heavily depends on the underlying architecture.
- C/C++: Moderately portable, with support for multiple platforms and architectures.
- Python: Highly portable, as it can run on various platforms without modifications.
Choosing the right programming language depends on the specific requirements of the project. Assembly offers unparalleled control over hardware, C/C++ strikes a balance between performance and abstraction, while Python prioritizes simplicity and ease of use. As developers, having knowledge of various languages empowers us to make informed decisions and craft the most suitable solutions.