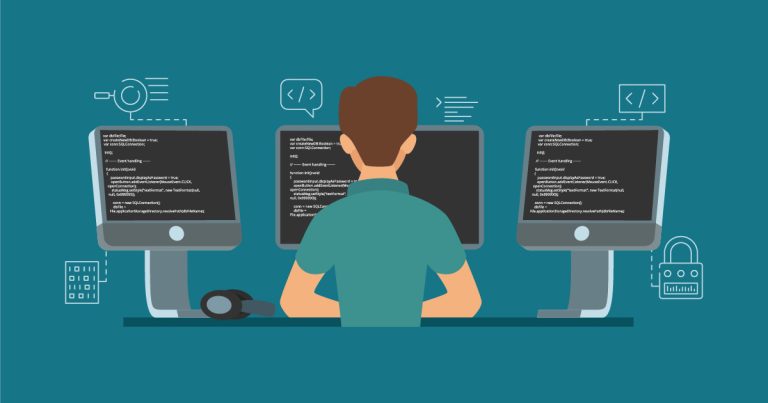
Exploring Object-Oriented Programming Principles in PHP
Object-Oriented Programming (OOP) is a powerful paradigm that enhances the organization, modularity, and reusability of code. PHP, a popular server-side scripting language, provides robust support for OOP, allowing developers to leverage its benefits. In this article, we will delve into the fundamental principles of OOP and their practical implementation in PHP.
Encapsulation
Encapsulation is the process of bundling data and related behaviors into a single entity called an object. It promotes data protection by controlling access to internal states and ensures that changes are made through well-defined methods. In PHP, encapsulation is achieved through access modifiers such as public
, private
, and protected
, which define the visibility and accessibility of properties and methods within a class.
Inheritance
Inheritance facilitates the creation of hierarchical relationships between classes, enabling the sharing of properties and methods. PHP supports single inheritance, where a child class inherits from a single parent class. By using the extends
keyword, a child class can inherit properties and methods from its parent class. Inheritance promotes code reuse and allows for the specialization and extension of existing classes.
Polymorphism
Polymorphism allows objects of different classes to be treated as instances of a common superclass, enabling interchangeable usage. PHP supports polymorphism through method overriding and interfaces. Method overriding allows a subclass to provide its own implementation of a method inherited from the parent class. Interfaces define a contract that classes can implement, ensuring consistency in behavior while allowing diverse implementations.
Abstraction
Abstraction involves capturing the essential features of an object while hiding unnecessary implementation details. In PHP, abstraction is achieved by using abstract classes and interfaces. Abstract classes provide a blueprint for derived classes, allowing them to implement common methods while leaving specific implementations to the derived classes. Interfaces define a set of methods that implementing classes must adhere to, promoting a standardized interface.
Composition
Composition involves building complex objects by combining simpler objects or components. It allows for greater flexibility and code reuse compared to inheritance. PHP supports composition through object aggregation and dependency injection. Object aggregation involves creating objects of other classes within a class, establishing a “has-a” relationship. Dependency injection allows the injection of dependencies into a class, enabling loose coupling and easy substitution of components.
Understanding and applying the principles of object-oriented programming in PHP can significantly improve code structure, maintainability, and scalability. By harnessing encapsulation, inheritance, polymorphism, abstraction, and composition, developers can create modular, reusable, and extensible applications. PHP’s robust OOP features empower developers to build complex systems while maintaining code readability and flexibility. Embracing these principles fosters clean, well-organized codebases, facilitating collaborative development and long-term project success.
In my next post we will explore some Best Practices for OOP in PHP. Best Practices for Object-Oriented Programming in PHP