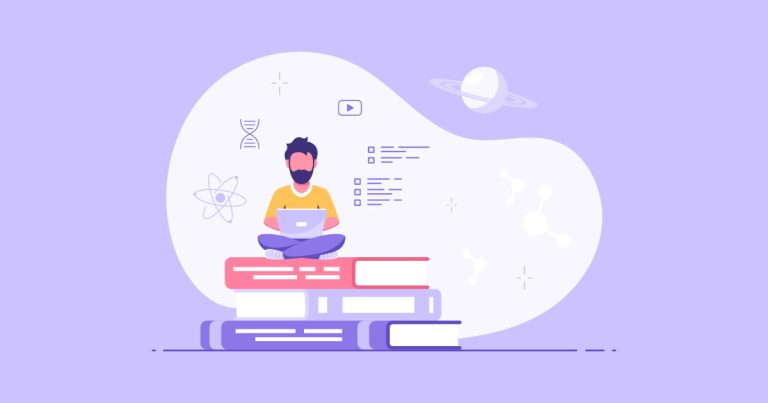
Demystifying Regular Expressions (Regex) – A Beginner’s Guide
Regular expressions, often referred to as regex, are powerful tools for pattern matching and text manipulation. Although they can appear intimidating at first glance, understanding the core concepts and syntax of regex will unlock a world of possibilities in text processing. In this beginner’s guide, we’ll demystify regex by breaking down its components and providing practical examples to help you grasp its fundamentals.
What is Regex?
Regex is a sequence of characters that define a search pattern. It enables you to match and manipulate text based on specific rules or patterns. Whether you need to validate email addresses, extract data, or find and replace text, regex can be your go-to tool.
Literal Matches
A literal match in regex allows you to find a specific sequence of characters. For example, the regex “hello” will match the word “hello” in any text.
Metacharacters
Metacharacters are special characters with predefined meanings in regex. Examples include:
.
(dot): Matches any single character except a newline.*
: Matches zero or more occurrences of the preceding character.+
: Matches one or more occurrences of the preceding character.?
: Matches zero or one occurrence of the preceding character.^
: Matches the start of a string.$
: Matches the end of a string.
Character Classes
Character classes allow you to match one character from a set of characters. For example, [aeiou]
will match any lowercase vowel.
Quantifiers
Quantifiers specify how many times a character or group should occur. For example, a{3}
matches exactly three consecutive ‘a’s.
Alternation
The |
symbol allows you to specify alternatives. For example, cat|dog
will match either “cat” or “dog.”
Grouping
Parentheses ()
are used to group parts of a regex together. They allow you to apply quantifiers to multiple characters or metacharacters.
Escaping Special Characters
To match a metacharacter as a literal character, you need to escape it using a backslash \
. For example, \.
will match a literal dot.
Modifiers
Modifiers allow you to control how the regex is applied. Common modifiers include:
i
: Case-insensitive matching.g
: Global matching (matches all occurrences).m
: Multi-line matching.
Practical Examples
- Validating an email address:
/^\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}\b$/
- Extracting URLs from text:
/https?:\/\/[^\s]+/
- Replacing multiple whitespaces:
/\s+/
Regular expressions might seem daunting at first, but with a solid understanding of their syntax and concepts, you can harness their power for various text processing tasks. As you practice and experiment with regex, you’ll gain confidence in crafting complex patterns to suit your specific needs. Remember to use online regex testers and documentation as valuable resources to fine-tune your regex expressions. Embrace the versatility of regex, and it will become an indispensable tool in your programming arsenal.