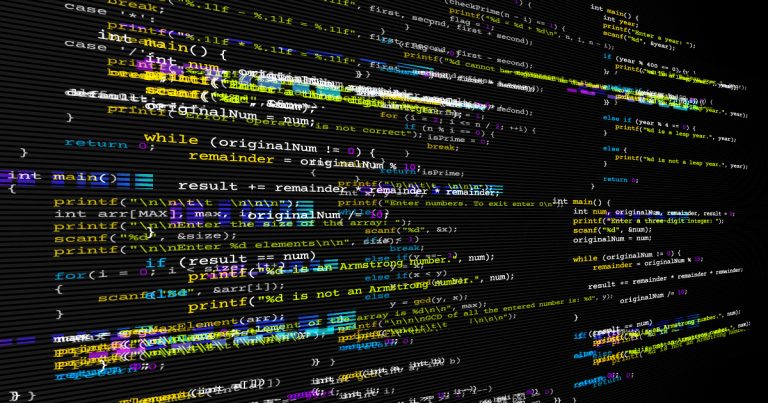
Best Practices for Object-Oriented Programming in PHP
When working with object-oriented programming in PHP, there are several best practices you can follow to ensure clean, maintainable, and efficient code. Here are some important practices to consider:
Follow SOLID Principles
- Single Responsibility Principle (SRP): Each class should have a single responsibility.
- Open/Closed Principle (OCP): Classes should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types.
- Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they don’t use.
- Dependency Inversion Principle (DIP): Depend upon abstractions, not concrete implementations.
Use Meaningful Naming
- Choose descriptive names for classes, methods, and variables that accurately convey their purpose.
- Follow standard naming conventions, such as using camelCase for methods and variables, and PascalCase for classes.
Maintain Code Readability
- Use proper indentation and formatting to enhance code readability.
- Include comments to explain complex logic, assumptions, and non-obvious functionality.
Apply Design Patterns
- Utilize well-known design patterns like Factory, Singleton, Observer, and Dependency Injection to address common software design challenges.
- Design patterns provide proven solutions that enhance code structure and maintainability.
Practice Modularity and Reusability
- Break down complex problems into smaller, cohesive classes and methods.
- Encapsulate related functionality within classes, following the single responsibility principle.
- Reuse existing code by leveraging inheritance, composition, and dependency injection.
Test-Driven Development (TDD)
- Write tests for your code before implementing the actual functionality.
- TDD promotes code quality, helps catch bugs early, and improves overall design.
Error Handling and Exception Management
- Use appropriate error handling techniques, such as try-catch blocks, to handle exceptions gracefully.
- Throw meaningful exceptions when encountering errors or exceptional conditions.
Optimize Performance
- Use efficient algorithms and data structures to minimize execution time and resource usage.
- Avoid unnecessary database queries or heavy computations within loops.
Document Your Code
- Provide clear and concise documentation for classes, methods, and significant code blocks.
- Document the purpose, input, output, and potential exceptions for each method.
Stay Updated
- Keep up with the latest PHP versions and features, as they often introduce improvements and optimizations.
- Stay informed about best practices and coding standards within the PHP community.
By following these best practices, you can ensure that your PHP codebase is maintainable, scalable, and adheres to industry standards, promoting collaboration and code quality.
Code Examples
Here are a few examples that illustrate best practices in object-oriented programming in PHP
Encapsulation and Meaningful Naming
class User {
private $username;
private $email;
public function __construct($username, $email) {
$this->username = $username;
$this->email = $email;
}
public function getUsername() {
return $this->username;
}
public function getEmail() {
return $this->email;
}
}
$user = new User("JohnDoe", "john.doe@example.com");
echo $user->getUsername(); // Output: JohnDoe
echo $user->getEmail(); // Output: john.doe@example.com
Inheritance
class Shape {
protected $color;
public function __construct($color) {
$this->color = $color;
}
public function getColor() {
return $this->color;
}
}
class Circle extends Shape {
private $radius;
public function __construct($color, $radius) {
parent::__construct($color);
$this->radius = $radius;
}
public function getArea() {
return pi() * $this->radius * $this->radius;
}
}
$circle = new Circle("Red", 5);
echo $circle->getColor(); // Output: Red
echo $circle->getArea(); // Output: 78.539816339745
Polymorphism
interface Animal {
public function makeSound();
}
class Dog implements Animal {
public function makeSound() {
echo "Woof!";
}
}
class Cat implements Animal {
public function makeSound() {
echo "Meow!";
}
}
$dog = new Dog();
$cat = new Cat();
$animals = [$dog, $cat];
foreach ($animals as $animal) {
$animal->makeSound();
}
// Output: Woof! Meow!
Abstraction and Composition
interface PaymentGateway {
public function processPayment($amount);
}
class PayPalGateway implements PaymentGateway {
public function processPayment($amount) {
echo "Processing payment of $" . $amount . " via PayPal.";
}
}
class Order {
private $paymentGateway;
public function __construct(PaymentGateway $paymentGateway) {
$this->paymentGateway = $paymentGateway;
}
public function processOrder($amount) {
// Process order logic
$this->paymentGateway->processPayment($amount);
// Additional order processing steps
}
}
$paypal = new PayPalGateway();
$order = new Order($paypal);
$order->processOrder(100);
// Output: Processing payment of $100 via PayPal.
These examples demonstrate key concepts and best practices in object-oriented programming using PHP, including encapsulation, inheritance, polymorphism, abstraction, and composition.