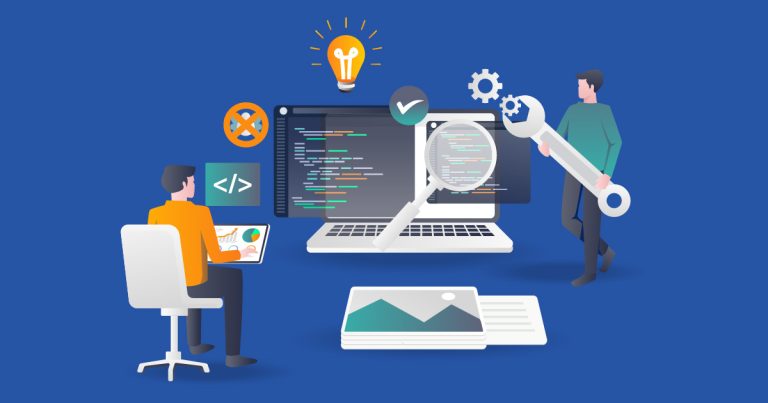
5 Advanced Tips for JavaScript: Level Up Your Coding Skills
JavaScript is a versatile programming language that empowers web developers to create dynamic and interactive web applications. To enhance your JavaScript prowess and write more efficient and elegant code, it’s beneficial to explore advanced tips and techniques. In this article, we will delve into five advanced tips for JavaScript, accompanied by code examples, to help you level up your coding skills.
1 – Utilize Destructuring Assignment
Destructuring assignment allows you to extract values from arrays or properties from objects into distinct variables, providing a concise and expressive way to work with complex data structures. Consider the following example:
// Array destructuring
const numbers = [1, 2, 3, 4, 5];
const [first, second, , fourth] = numbers;
console.log(first, second, fourth); // Output: 1 2 4
// Object destructuring
const person = { name: 'John', age: 30, city: 'New York' };
const { name, age } = person;
console.log(name, age); // Output: John 30
Destructuring assignment enhances code readability and simplifies variable assignment from arrays and objects.
2 – Take Advantage of Promises and Async/Await
Asynchronous programming is essential for handling time-consuming operations efficiently. Promises and async/await offer powerful mechanisms to work with asynchronous code. Consider the following example using async/await:
function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
resolve('Data fetched successfully!');
}, 2000);
});
}
async function processRequest() {
console.log('Processing request...');
const result = await fetchData();
console.log(result);
}
processRequest();
Promises and async/await enable writing more readable and sequential asynchronous code, avoiding callback hell and enhancing error handling.
3 – Implement Memoization
Memoization is a technique that allows you to cache the results of expensive function calls, improving performance by avoiding redundant computations. Here’s an example of a memoization function:
function memoize(func) {
const cache = {};
return function (...args) {
const key = JSON.stringify(args);
if (key in cache) {
return cache[key];
}
const result = func.apply(this, args);
cache[key] = result;
return result;
};
}
function fibonacci(n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
const memoizedFibonacci = memoize(fibonacci);
console.log(memoizedFibonacci(10)); // Output: 55
Memoization can significantly improve the performance of recursive or computationally expensive functions by reusing cached results for the same input.
4 – Apply Currying for Function Composition
Currying is a technique that transforms a function with multiple arguments into a sequence of functions, each taking a single argument. It allows for more flexible function composition and reusability. Here’s an example:
function add(x) {
return function (y) {
return x + y;
};
}
const addFive = add(5);
console.log(addFive(3)); // Output: 8
console.log(addFive(7)); // Output: 12
Currying facilitates the creation of specialized functions by partially applying arguments, making code more modular and flexible.
5 – Explore Functional Programming Concepts
Functional programming concepts promote writing more declarative, immutable, and concise code. JavaScript provides several built-in functions to support functional programming, such as map
, filter
, reduce
, and forEach
. Here’s an example using map
and arrow functions:
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map((num) => num ** 2);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Functional programming techniques encourage writing code that is easier to reason about and test, with fewer side effects and mutable state.
By applying these advanced tips and techniques in JavaScript, you can enhance your coding skills, improve code quality, and boost the efficiency of your applications. Destructuring assignment, promises and async/await, memoization, currying, and functional programming concepts offer powerful tools to write cleaner, more concise, and performant code. Embrace these techniques to unlock new possibilities and take your JavaScript coding to the next level.