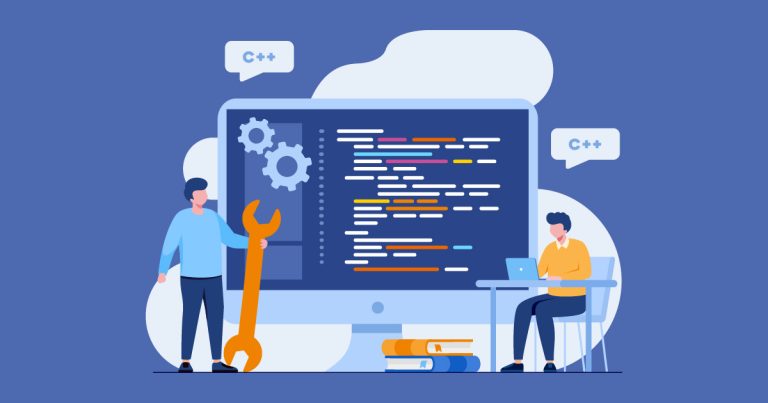
5 Advanced Tips and Tricks in C++
C++ is a powerful and versatile programming language that has been widely used for decades in various domains, including system programming, game development, and more. While C++ can be challenging to master, it offers numerous advanced techniques that can significantly improve code efficiency, readability, and maintainability. In this article, we will explore five advanced tips and tricks in C++ that can help developers write cleaner, more robust, and optimized code.
1 – Smart Pointers for Memory Management
C++11 introduced smart pointers, which are a safer and more efficient way to manage dynamic memory allocation compared to traditional raw pointers. The three primary types of smart pointers are std::unique_ptr
, std::shared_ptr
, and std::weak_ptr
.
std::unique_ptr
represents exclusive ownership of an object and ensures that only one pointer can point to the allocated memory. It automatically deletes the object when it goes out of scope, reducing the chances of memory leaks.
std::shared_ptr
allows multiple pointers to share ownership of the same object. The object will be deleted only when the last shared_ptr
pointing to it goes out of scope. This feature is especially useful when dealing with complex data structures and avoiding premature deallocation.
std::weak_ptr
is used in conjunction with std::shared_ptr
to break circular references, avoiding memory leaks. It provides a non-owning reference to the object held by std::shared_ptr
without increasing the reference count.
2 – Move Semantics for Efficient Resource Management
Move semantics, introduced in C++11, allow the efficient transfer of resources, such as memory allocations or ownership of dynamically allocated objects, between objects. This feature significantly reduces the number of expensive deep copies and improves the performance of programs working with heavy objects.
By implementing move constructors and move assignment operators, developers can transfer ownership of resources rather than making expensive copies. This technique is particularly beneficial for large data structures, such as containers, which can be moved without duplicating their content.
3 – Lambda Functions for Concise Code
Lambda functions, introduced in C++11, provide a concise and efficient way to create small, inline functions, also known as closures. Lambdas are particularly useful when we need a simple function that might only be used in a specific context.
The syntax for a lambda function is as follows:
[ captures ] ( parameters ) -> return_type {
// Function body
}
Here, captures
can be used to capture variables from the surrounding scope by reference or by value.
Using lambda functions can lead to more readable and maintainable code, especially when working with standard algorithms like std::for_each
, std::transform
, or std::sort
.
4 – Custom Memory Allocators for Performance Optimization
By default, C++ allocates memory using the global operator new
and deallocates using operator delete
. While this approach works well for general purposes, it might not be the most efficient for specific use cases.
Developers can create custom memory allocators tailored to their application’s needs, potentially leading to significant performance improvements. For instance, using a memory pool allocator can reduce the overhead of frequent memory allocations and deallocations, resulting in faster and more efficient code execution.
5 – Variadic Templates for Flexibility
Variadic templates, introduced in C++11, allow developers to create functions and classes that take a variable number of arguments. This feature enhances code flexibility and enables the implementation of generic and reusable components.
The syntax for defining a variadic template function is as follows:
template<typename... Args>
void myFunction(Args... args) {
// Function body
}
Variadic templates are extensively used in the Standard Template Library (STL), making it possible to create container classes that can hold different types of elements or to write algorithms that work with an arbitrary number of arguments.
C++ is a powerful language that provides developers with numerous advanced features to write efficient, maintainable, and flexible code. By incorporating smart pointers, move semantics, lambda functions, custom memory allocators, and variadic templates into their codebase, programmers can take their C++ skills to the next level and build high-performance applications across various domains. Embracing these advanced tips and tricks will undoubtedly contribute to becoming a more proficient C++ developer.