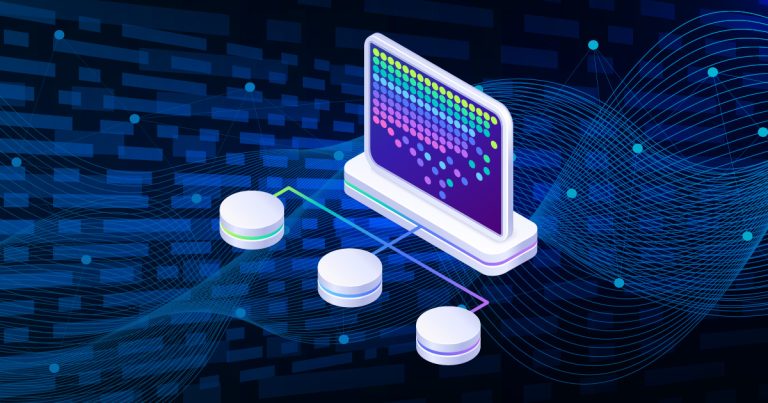
5 Advanced and Must-Use Tips and Tricks for PDO in PHP
PHP Data Objects (PDO) is a powerful database abstraction layer that provides a consistent interface for interacting with various databases. If you’re already familiar with PDO basics, it’s time to explore some advanced tips and tricks to unleash the full potential of PDO in your PHP applications. In this article, we’ll cover five advanced techniques that will elevate your database operations to the next level.
1 – Using Transactions for Atomicity
Transactions ensure the atomicity of database operations, meaning they either all succeed, or none of them do. This prevents data inconsistencies and maintains the integrity of your data. Use the beginTransaction()
, commit()
, and rollback()
methods to handle transactions in PDO:
try {
$pdo->beginTransaction();
// Perform multiple database operations
$pdo->query('INSERT INTO users (name, email) VALUES ("John Doe", "john@example.com")');
$pdo->query('UPDATE balance SET amount = amount - 100 WHERE user_id = 1');
$pdo->commit();
} catch (PDOException $e) {
$pdo->rollback();
echo 'Transaction failed: ' . $e->getMessage();
}
2 – Prepared Statements with Named Placeholders
PDO supports both anonymous and named placeholders in prepared statements. Named placeholders make your code more readable and maintainable, especially when dealing with complex queries:
$name = 'John Doe';
$email = 'john@example.com';
$stmt = $pdo->prepare('INSERT INTO users (name, email) VALUES (:name, :email)');
$stmt->bindParam(':name', $name);
$stmt->bindParam(':email', $email);
$stmt->execute();
3 – Leveraging Fetch Modes
PDO provides various fetch modes to control how result data is returned. The default fetch mode is PDO::FETCH_BOTH
, which returns an array with both numeric and associative keys. However, you can use other modes, such as PDO::FETCH_ASSOC
, PDO::FETCH_OBJ
, or custom fetch styles:
// Fetching as associative array
$stmt = $pdo->query('SELECT name, email FROM users');
$results = $stmt->fetchAll(PDO::FETCH_ASSOC);
// Fetching as objects
$stmt = $pdo->query('SELECT name, email FROM users');
$results = $stmt->fetchAll(PDO::FETCH_OBJ);
4 – Handling BLOB Data
Binary Large Objects (BLOB) are used to store large data, such as images or documents, in databases. When dealing with BLOB data, use prepared statements with the PDO::PARAM_LOB
parameter type to ensure correct data handling:
$image = file_get_contents('path/to/image.jpg');
$stmt = $pdo->prepare('INSERT INTO images (name, data) VALUES (:name, :data)');
$stmt->bindParam(':name', $imageName);
$stmt->bindParam(':data', $image, PDO::PARAM_LOB);
$stmt->execute();
5 – Custom Error Handling with PDOException
Custom error handling can help you better manage PDO exceptions and log errors appropriately. Implement a custom error handler with set_exception_handler()
to catch and handle PDO-related exceptions:
function customExceptionHandler(PDOException $e) {
// Log the exception or perform custom error handling
echo 'Custom Error Handler: ' . $e->getMessage();
}
set_exception_handler('customExceptionHandler');
// Perform PDO operations
// If an exception occurs, it will be caught by the customExceptionHandler function
By utilizing these advanced tips and tricks for PDO in PHP, you can enhance the security, performance, and maintainability of your database operations. Remember to refer to the official PHP documentation and explore further in-depth resources to deepen your understanding of PDO and refine your skills.